For one of my Ember.js apps, I have a bit of a too complex Form flow. While I’m working on simplifying frontend, I wanted a way to easily log validation errors that users received. In addition to debugging, this helps me improve the labels and instructions on the form itself.
Backend in this case if Django Request Framework driven with JSON API. The idea is to log all validation errors and redirect them to Sentry in Debug mode:
First we declare a custom DRF Exception handler the uses JSON API exception handler and copies the data to sentry:
// exceptions.py
from rest_framework_json_api.exceptions import exception_handler
from raven.contrib.django.raven_compat.models import client
from app.serializers import SubmissionSerializer
def custom_drf_exception_handler(exc, context):
response = exception_handler(exc, context)
if isinstance(context.get('view').get_serializer_class(), SubmissionSerializer):
client.captureMessage('Form Submission Validation Error', level='debug', extra=exc.get_full_details())
return response
And we also have to configure DRF to re-route errors through it:
// settings.py
REST_FRAMEWORK = {
..
'EXCEPTION_HANDLER': 'app.exceptions.custom_drf_exception_handler',
..
}
And that’s it. The end result is that when serialization error is triggered, we now get a nice error log in Sentry:
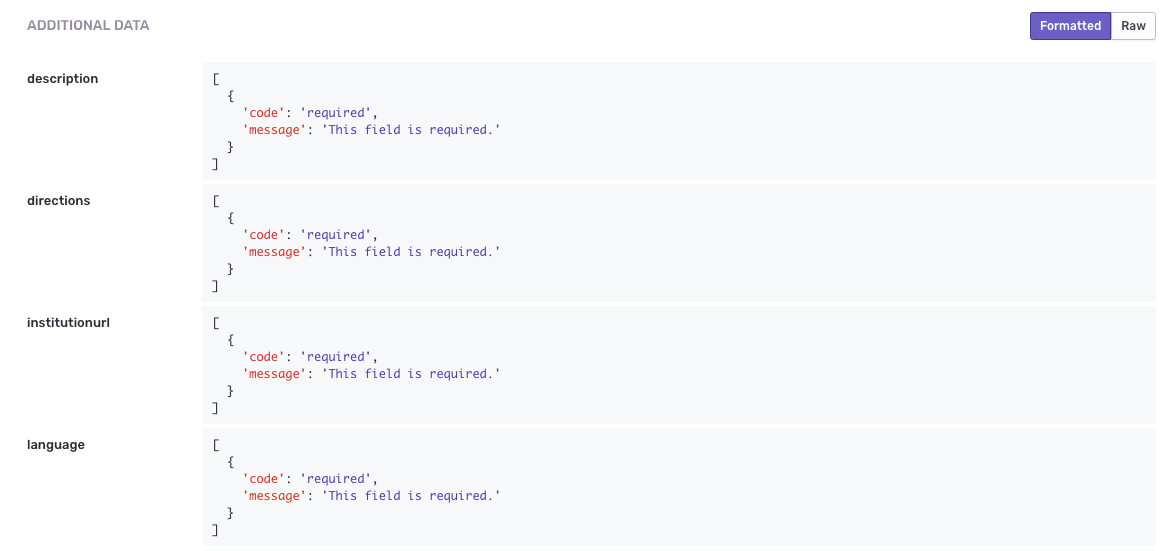